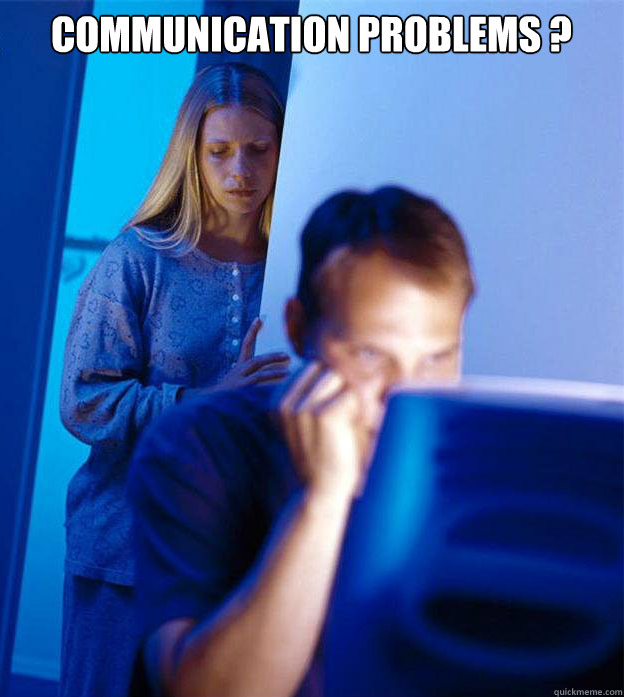
import javax.jms.Connection; import org.apache.activemq.spring.ActiveMQConnectionFactory; ActiveMQConnectionFactory connectionFactory = new ActiveMQConnectionFactory(); connectionFactory.setBrokerURL("tcp://localhost:61616"); connection = connectionFactory.createConnection(); connection.start(); Session session = connection.createSession(false, Session.AUTO_ACKNOWLEDGE); Destination destination = session.createTopic("Testtopic"); MessageProducer producer = session.createProducer(destination); producer.setDeliveryMode(DeliveryMode.NON_PERSISTENT); String text = "Message String"; TextMessage message = session.createTextMessage(text); producer.send(message); session.close();
import javax.jms.Connection; import org.apache.activemq.spring.ActiveMQConnectionFactory; ActiveMQConnectionFactory connectionFactory = new ActiveMQConnectionFactory(); connectionFactory.setBrokerURL("tcp://localhost:61616"); Connection connection = connectionFactory.createConnection(); connection.start(); Session session = connection.createSession(false, Session.AUTO_ACKNOWLEDGE); MessageConsumer consumer = session.createConsumer(session.createTopic("Testtopic")); consumer.setMessageListener(new MessageListener());
require 'amqp' AMQP.start(:host => 'localhost') do |connection| AMQP::Channel.new(connection) do |channel, open_ok| channel.on_error do |channel, channel_close| puts "Error #{channel_close.reply_code}: #{channel_close.reply_text}" end channel.queue('/queue/test_queue', :durable => true) do |queue, declare_ok| channel.default_exchange.publish 'Message String', :routing_key => queue.name, :persistent => true EventMachine.add_timer(0.5) { connection.disconnect { EventMachine.stop } } end end end
require 'amqp' AMQP.start(:host => 'localhost') do |connection| AMQP::Channel.new(connection) do |channel, open_ok| channel.on_error(&method(:handle_channel_exception)) channel.queue('/queue/test_queue', :durable => true) do |queue, declare_ok| queue.subscribe(:ack => true) do |header, message| puts "Received #{message}" header.ack if (message == 'QUIT') queue.unsubscribe connection.disconnect { EventMachine.stop } end end end end end
require 'stomp' client = Stomp::Client.new(:host => 'localhost') client.publish '/queue/test_queue', 'Message String', :persistent => true client.close
require 'stomp' client = Stomp::Client.new(:host => 'localhost') client.subscribe '/queue/test_queue', :ack => :client do |message| puts "Received #{message}" client.acknowledge(message) client.close if (message == 'QUIT') end client.join
require 'ffi-rzmq' context = ZMQ::Context.new 1 sender = context.socket ZMQ::PUSH sender.connect('tcp://127.0.0.1:5555') sender.send_string('Message String') sender.close
require 'ffi-rzmq' context = ZMQ::Context.new 1 receiver = context.socket ZMQ::PULL receiver.bind('tcp://127.0.0.1:5555') message = '' receiver.recv_string(message) puts "Received #{message}" receiver.close